【SSM框架】为集合类型属性赋值
🍓个人主页:个人主页
🍒系列专栏:SSM框架
目录
1.为集合类型属性赋值
①为List集合类型属性赋值
②为Map集合类型属性赋值
2.p命名空间
3.引入外部属性文件
1.为集合类型属性赋值
①为List集合类型属性赋值
在
Clazz
类中添加以下代码:
private List<Student> students;
public List<Student> getStudents() {
return students;
}
public void setStudents(List<Student> students) {
this.students = students;
}
方法1:配置bean:
若为Set集合类型属性赋值,只需要将其中的list标签改为set标签即可
<bean id="clazzTwo" class="com.atguigu.spring.pojo.Clazz">
<property name="clazzId" value="1"></property>
<property name="clazzName" value="计算机科学与技术"></property>
<property name="students">
<list>
<ref bean="studentOne"></ref>
<ref bean="studentTwo"></ref>
<ref bean="studentThree"></ref>
</list>
</property>
</bean>
<bean id="studentOne" class="com.atguigu.spring.pojo.Student">
<property name="id" value="1001"></property>
<property name="name" value="赵1"></property>
<property name="age" value="22"></property>
<property name="sex" value="女"></property>
<property name="hobbies">
<array>
<value>吃饭</value>
<value>睡觉</value>
<value>打豆豆</value>
</array>
</property>
</bean>
<bean id="studentTwo" class="com.atguigu.spring.pojo.Student">
<property name="id" value="1002"></property>
<property name="name" value="赵2"></property>
<property name="age" value="24"></property>
<property name="sex" value="女"></property>
<property name="hobbies">
<array>
<value>吃饭</value>
<value>睡觉</value>
<value>打豆豆</value>
</array>
</property>
</bean>
<bean id="studentThree" class="com.atguigu.spring.pojo.Student">
<property name="id" value="1004"></property>
<property name="name" value="赵3"></property>
<property name="age" value="21"></property>
<property name="sex" value="女"></property>
<property name="hobbies">
<array>
<value>吃饭</value>
<value>睡觉</value>
<value>打豆豆</value>
</array>
</property>
</bean>
测试:
@org.junit.Test
public void testHelloWorld(){
ApplicationContext ac = new ClassPathXmlApplicationContext("applicationContext.xml");
Clazz clazzTwo = ac.getBean("clazzTwo", Clazz.class);
System.out.println(clazzTwo);
方法2:配置一个集合类型的bean,需要使用util 的约束-
<bean id="clazzTwo" class="com.atguigu.spring.pojo.Clazz">
<property name="clazzId" value="1"></property>
<property name="clazzName" value="计算机科学与技术"></property>
<property name="students" ref="studentsList">
</property>
</bean>
<util:list id="studentsList">
<ref bean="studentOne"></ref>
<ref bean="studentTwo"></ref>
<ref bean="studentThree"></ref>
</util:list>
效果一样的:
②为Map集合类型属性赋值
创建教师类Teacher:
public class Teacher {
private Integer tid;
private String tname;
public Teacher() {
}
public Teacher(Integer tid, String tname) {
this.tid = tid;
this.tname = tname;
}
public Integer getTid() {
return tid;
}
public void setTid(Integer tid) {
this.tid = tid;
}
public String getTname() {
return tname;
}
public void setTname(String tname) {
this.tname = tname;
}
@Override
public String toString() {
return "Teacher{" +
"tid=" + tid +
", tname='" + tname + '\'' +
'}';
}
}
在Student类中添加以下代码:
private Map<String, Teacher> teacherMap;
public Map<String, Teacher> getTeacherMap() {
return teacherMap;
}
public void setTeacherMap(Map<String, Teacher> teacherMap) {
this.teacherMap = teacherMap;
}
方法1:配置bean(
引用集合类型的
bean
):
<bean id="student" class="com.atguigu.spring.pojo.Student">
<property name="id" value="1004"></property>
<property name="name" value="赵六"></property>
<property name="age" value="26"></property>
<property name="sex" value="女"></property>
<property name="clazz">
<!-- 在一个bean中再声明一个bean就是内部bean -->
<!-- 内部bean只能用于给属性赋值,不能在外部通过IOC容器获取,因此可以省略id属性 -->
<bean id="clazzInner" class="com.atguigu.spring.pojo.Clazz">
<property name="clazzId" value="2"></property>
<property name="clazzName" value="软件工程"></property>
</bean>
</property>
<property name="hobbies">
<array>
<value>吃饭</value>
<value>睡觉</value>
<value>打豆豆</value>
</array>
</property>
<property name="teacherMap">
<map>
<entry key="1" value-ref="teacherOne"></entry>
<entry key="2" value-ref="teacherTwo"></entry>
</map>
</property>
</bean>
<bean id="teacherOne" class="com.atguigu.spring.pojo.Teacher">
<property name="tid" value="11111"></property>
<property name="tname" value="小王"></property>
</bean>
<bean id="teacherTwo" class="com.atguigu.spring.pojo.Teacher">
<property name="tid" value="22222"></property>
<property name="tname" value="小李"></property>
</bean>
测试:
public void testHelloWorld(){
ApplicationContext ac = new ClassPathXmlApplicationContext("applicationContext.xml");
Student student = ac.getBean("student", Student.class);
System.out.println(student);
}
方法2:配置bean(util:map):
<bean id="student" class="com.atguigu.spring.pojo.Student">
<property name="id" value="1004"></property>
<property name="name" value="赵六"></property>
<property name="age" value="26"></property>
<property name="sex" value="女"></property>
<property name="clazz">
<!-- 在一个bean中再声明一个bean就是内部bean -->
<!-- 内部bean只能用于给属性赋值,不能在外部通过IOC容器获取,因此可以省略id属性 -->
<bean id="clazzInner" class="com.atguigu.spring.pojo.Clazz">
<property name="clazzId" value="2"></property>
<property name="clazzName" value="软件工程"></property>
</bean>
</property>
<property name="hobbies">
<array>
<value>吃饭</value>
<value>睡觉</value>
<value>打豆豆</value>
</array>
</property>
<property name="teacherMap" ref="teacherMap">
</property>
</bean>
<util:map id="teacherMap">
<entry key="1" value-ref="teacherOne"></entry>
<entry key="2" value-ref="teacherTwo"></entry>
</util:map>
2.p命名空间
引入p命名空间后,可以通过以下方式为bean的各个属性赋值
<bean id="studentT" class="com.atguigu.spring.pojo.Student" p:name="李王" p:id="1009" p:teacherMap-ref="teacherMap">
</bean>
3.引入外部属性文件
①加入依赖
<!-- MySQL驱动 -->
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.16</version>
</dependency>
<!-- 数据源 -->
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>druid</artifactId>
<version>1.0.31</version>
</dependency>
②创建外部属性文件
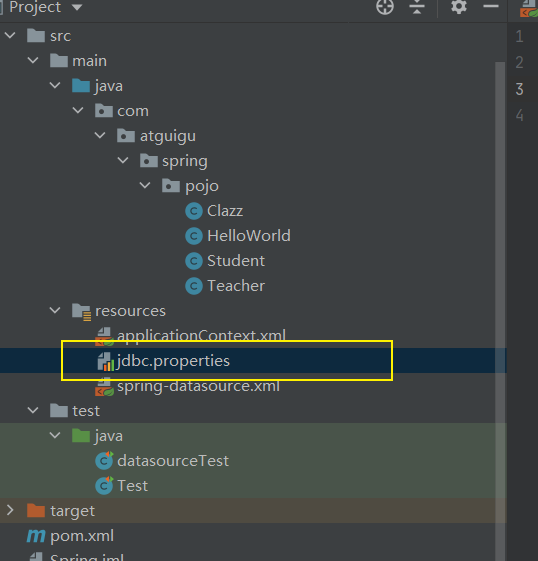
jdbc.driver=com.mysql.cj.jdbc.Driver
jdbc.url=jdbc:mysql://localhost:3306/ssm? serverTimezone=UTC
jdbc.username=root
jdbc.password=root
③引入属性文件
<context:property-placeholder location="jdbc.properties"></context:property-placeholder>
④配置
bean
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context-4.2.xsd">
<context:property-placeholder location="jdbc.properties"></context:property-placeholder>
<bean id="dataSource" class="com.alibaba.druid.pool.DruidDataSource">
<property name="url" value="${jdbc.url}"/>
<property name="driverClassName" value="${jdbc.driver}"/>
<property name="username" value="${jdbc.username}"/>
<property name="password" value="${jdbc.password}"/>
</bean>
</beans>
⑤测试
public void test(){
ClassPathXmlApplicationContext ioc = new ClassPathXmlApplicationContext("spring-datasource.xml");
DruidDataSource bean = ioc.getBean(DruidDataSource.class);
try {
System.out.println(bean.getConnection());
} catch (SQLException throwables) {
throwables.printStackTrace();
}}