1.The Graphics View Architecture(图形视图框架)
--The Scene(场景)
--The View(视图)
--The Item(对象)
1.The Graphics View Architecture(图形视图框架)
Graphics View provides an item-based approach to model-view programming, much like InterView's convenience classes QTableView, QTreeView and QListView. Several views can observe a single scene, and the scene contains items of varying geometric shapes.
图形视图提供了基于图像对象的方式来实现model-view(模型视图)的编程模式,这个很像InterView的辅助类QTableView, QTreeView 和QListView。不同的视图能够显示一个单一场景,而且这个场景包含了不同的几何形状对象。
1.1The Scene(场景)
QGraphicsScene provides the Graphics View scene. The scene has the following responsibilities:
——Providing a fast interface for managing a large number of items
——Propagating events to each item
——Managing item state, such as selection and focus handling
——Providing untransformed rendering functionality; mainly for printing
The scene serves as a container for QGraphicsItem objects. Items are added to the scene by calling QGraphicsScene::addItem(), and then retrieved by calling one of the many item discovery functions. QGraphicsScene::items() and its overloads return all items contained by or intersecting with a point, a rectangle, a polygon or a general vector path. QGraphicsScene::itemAt() returns the topmost item at a particular point. All item discovery functions return the items in descending stacking order (i.e., the first returned item is topmost, and the last item is bottom-most).
QGraphicsScene提供了图形视图的场景管理器。场景管理器有如下职责:
——提供一个用于管理大量对象的快速接口
——将事件传递到每个对象上
——管理对象的状态,例如,搜索和集中处理
——提供未进行变换的渲染功能,主要用于打印
场景管理器是图形对象 QGraphicsItem的容器。调用QGraphicsScene::addItem()将对象添加到场景中,然后就可以通过调用 其中的一个对象查找方法来重新取回。不同的查找函数来查找其中的图形对象。QGraphicsScene::items()函数及其重载函数可以返回所有对象,包含点、矩形、多边形或普通的矢量路径等所有对象。QGraphicsScene::itemAt()返回在指定点位置上最上面的对象。所有的对象查找函数都返回按照堆栈递减排列顺序的对象(即第一个返回的对象是最顶层,和最后一个项目是最底层的对象)。
QGraphicsScene scene;
QGraphicsRectItem *rect = scene.addRect(QRectF(0, 0, 100, 100));
QGraphicsItem *item = scene.itemAt(50, 50);
// item == rect
QGraphicsScene's event propagation architecture schedules scene events for delivery to items, and also manages propagation between items. If the scene receives a mouse press event at a certain position, the scene passes the event on to whichever item is at that position.
QGraphicsScene的事件传递机制负责将场事件景传递给图形对象,同时也管理对象之间的事件传递。如果场景在某个位置
得到鼠标按下的事件,就将该事件传递给在这个位置上的对象。
QGraphicsScene also manages certain item states, such as item selection and focus. You can select items on the scene by calling QGraphicsScene::setSelectionArea(), passing an arbitrary shape. This functionality is also used as a basis for rubberband selection in QGraphicsView. To get the list of all currently selected items, call QGraphicsScene::selectedItems(). Another state handled by QGraphicsScene is whether or not an item has keyboard input focus. You can set focus on an item by calling QGraphicsScene::setFocusItem() or QGraphicsItem::setFocus(), or get the current focus item by calling QGraphicsScene::focusItem().
QGraphicsScene同时也还管理某些对象的状态,例如对象的选中和focus状态。你可以在场景中选取的对象通过调用QGraphicsScene::setSelectionArea(),传递给该对象一个任意形状。此功能也是 QGraphicsView 中拉框选择( rubber band)的基础。通过调用 QGraphicsScene::selectedItems()可以获取当前选择的所有对象列表。另外一种通过 QGraphicsScene 来管理处理的状态是:一个对象是否拥有键盘输入焦点。 .你可以调用 QGraphicsScene::setFocusItem ()或者 QGraphicsItem::setFocus ()将键盘焦点切换到对于的图形对象上,或者通过 QGraphicsScene::focusItem ()获取当前的焦点对象。
Finally, QGraphicsScene allows you to render parts of the scene into a paint device through the QGraphicsScene::render() function. You can read more about this in the Printing section later in this document.
最后, QGraphicsScene 允许你通过 QGraphicsScene::render ()将部分场景绘制到 绘制设备上。你可以在本文档中关于“打印”的章节了解更多关于这一点的知识。
1.2The View(视图)
QGraphicsView provides the view widget, which visualizes the contents of a scene. You can attach several views to the same scene, to provide several viewports into the same data set. The view widget is a scroll area, and provides scroll bars for navigating through large scenes. To enable OpenGL support, you can set a QGLWidget as the viewport by calling QGraphicsView::setViewport().
QGraphicsView 提供了视图组件,将场景中的内容显示出来。你可以用几个不同的视图来观察同一个场景,从而实现对于同一数据集的不同观察。该视图组件同时也是滚动型的区域,为大场景提供滚动条。如果要启用 OpenGL支持,可调用 QGraphicsView::setViewport 将 QGLWidget 设置为其观察口。
QGraphicsScene scene;
myPopulateScene(&scene);
QGraphicsView view(&scene);
view.show();
The view receives input events from the keyboard and mouse, and translates these to scene events (converting the coordinates used to scene coordinates where appropriate), before sending the events to the visualized scene.
视图接收键盘和鼠标的输入事件,并将这些消息转换成场景事件(同时将视图坐标转换为场景坐标),然后将事件发送给可见视图。
Using its transformation matrix, QGraphicsView::transform(), the view can transform the scene's coordinate system. This allows advanced navigation features such as zooming and rotation. For convenience, QGraphicsView also provides functions for translating between view and scene coordinates: QGraphicsView::mapToScene() and QGraphicsView::mapFromScene().
通过使用变换矩阵 QGraphicsView::transform ,视图可以对场景的坐标系统进行变换。这个方法可以实现缩放、旋转等高级功能。为了方便起见, QGraphicsView同时也提供了在视图坐标和场景坐标之间变化的函数: QGraphicsView::mapToScene () 和 QGraphicsView::mapFromScene ()。
1.3The Item(对象)
——Mouse press, move, release and double click events, as well as mouse hover events, wheel events, and context menu events.
——Keyboard input focus, and key events
——Drag and drop
——Grouping, both through parent-child relationships, and with QGraphicsItemGroup
——Collision detection
与 QGraphicsView 类似,在局部坐标系下的对象,也提供了很多对象和场景之间的绘图坐标函数。和 QGraphicsView 一样,它同时还可以通过矩阵QGraphicsItem::transform()来变换其自身的坐标系统。这点对于单个图形对象的旋转和缩放非常有用。
Items can contain other items (children). Parent items' transformations are inherited by all its children. Regardless of an item's accumulated transformation, though, all its functions (e.g., QGraphicsItem::contains(), QGraphicsItem::boundingRect(), QGraphicsItem::collidesWith()) still operate in local coordinates.
对象可以包含其他对象(子对象)。父对象的变换可以被他的子对象继承。不管一个对象累积了多少变换, 它的 函数QGraphicsItem::collidesWith()仍然会在局部坐标系下进行计算。
QGraphicsItem supports collision detection through the QGraphicsItem::shape() function, and QGraphicsItem::collidesWith(), which are both virtua l functions. By returning your item's shape as a local coordinate QPainterPath from QGraphicsItem::shape(), QGraphicsItem will handle all collision detection for you. If you want to provide your own collision detection, however, you can reimplement QGraphicsItem::collidesWith().
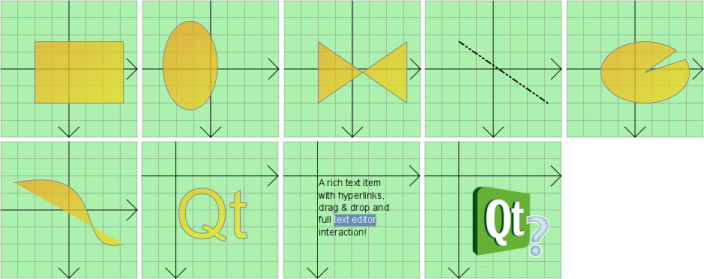