2019独角兽企业重金招聘Python工程师标准>>>
NSOperation Class Reference
NSOperation 類參考
本文PDF版本:
http://db.tt/A1xLPVXq。(排版較佳)
轉載請註明出處,謝謝。

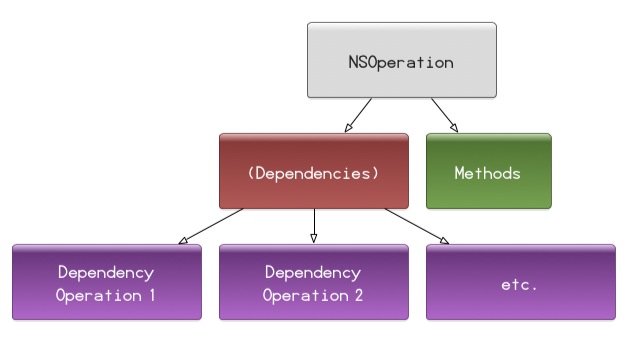
原文網址:http://developer.apple.com/documentation/Cocoa/Reference/NSOperation_class/Reference/Reference.html。(Last updated: 2012-01-09)
本文網址:http://my.oschina.net/KSHime37。(2012-09-18)
Overview
概論
The NSOperation class is an abstract class you use to encapsulate the code and data associated with a single task. Because it is abstract, you do not use this class directly but instead subclass or use one of the system-defined subclasses (NSInvocationOperation or NSBlockOperation) to perform the actual task. Despite being abstract, the base implementation of NSOperation does include significant logic to coordinate the safe execution of your task. The presence of this built-in logic allows you to focus on the actual implementation of your task, rather than on the glue code needed to ensure it works correctly with other system objects.
工作物件(NSOperation)是一個抽象的類,可以將程式碼和數據封裝成任務以便執行使用。因為其為抽象類,所以必需以子類繼承使用,或者使用系統提供的子類(NSInvocationOperation或NSBlockOperation),以執行實際的工作。儘管其為抽象類,但其也有包含了基本的邏輯及安全的執行以供使用。因為內建邏輯的存在,可以專心的為其實現實際的工作,而不是以混亂的程式碼使其與其他系統物件準確的工作。
An operation object is a single-shot object—that is, it executes its task once and cannot be used to execute it again. You typically execute operations by adding them to an operation queue (an instance of the NSOperationQueue class). An operation queue executes its operations either directly, by running them on secondary threads, or indirectly using the libdispatch library (also known as Grand Central Dispatch). For more information about how queues execute operations, see NSOperationQueue Class Reference.
一個工作物件是一個一次性的物件—也就是說,其只能被執行一次,不能重復執行。典型的使用方法是將其添加到管理器(NSOperationQueue的實例)的隊列中。管理器會準確的執行之,將其執行於其他的線程中,或者間接使用libdispatch庫(也就是GCD)執行之。更多有關管理器執行隊列工作的細節,請見NSOperationQueue Class Reference。
If you do not want to use an operation queue, you can execute an operation yourself by calling its start method directly from your code. Executing operations manually does put more of a burden on your code, because starting an operation that is not in the ready state triggers an exception. The isReady method reports on the operation’s readiness.
如果不使用管理器來執行,必需自行於程式碼中呼叫工作物件的-(void)start方法。手動執行工作物件會使程式碼的負擔加重,因為其在非就緒狀態下是不會執行的,而工作物件的-(BOOL)isReady方法會回報其就緒狀態。
Operation Dependencies
工作物件的從屬關係
Dependencies are a convenient way to execute operations in a specific order. You can add and remove dependencies for an operation using the addDependency: and removeDependency: methods. By default, an operation object that has dependencies is not considered ready until all of its dependent operation objects have finished executing. Once the last dependent operation finishes, however, the operation object becomes ready and able to execute.
從屬關係是用於決定工作執行順序的便利方法。可以使用工作物件的addDependency:方法及removeDependency:方法來添加或移除從屬關係。默認情況下,一個工作物件在其依賴的工作物件完成前是不會被執行的。直到其依賴的最後一個工作物件完成,其才會準備就緒,可以開始執行。
The dependencies supported by NSOperation make no distinction about whether a dependent operation finished successfully or unsuccessfully. (In other words, canceling an operation similarly marks it as finished.) It is up to you to determine whether an operation with dependencies should proceed in cases where its dependent operations were cancelled or did not complete their task successfully. This may require you to incorporate some additional error tracking capabilities into your operation objects.
工作物件(NSOperation)提供的從屬關係不會判斷其依賴之工作物件是否成功的執行完工作(取消工作物件也同樣代表完成)。這就需要自行追蹤相關訊息以判斷其從屬的對象是否被取消或者成功完成。
KVO-Compliant Properties
鍵值觀察的屬性
The NSOperation class is key-value coding (KVC) and key-value observing (KVO) compliant for several of its properties. As needed, you can observe these properties to control other parts of your application. The properties you can observe include the following:
工作物件(NSOperation)的部分屬性遵循鍵值編程(KVC)以及鍵值觀察(KVO)。如果有需要,可以透過觀察這些屬性來控制程式其他的部分。以下是可以觀察的屬性:
isCancelled - read-only property
(是否取消,只讀)
isConcurrent - read-only property
(是否並行,只讀)
isExecuting - read-only property
(是否執行中,只讀)
isFinished - read-only property
(是否完成,只讀)
isReady - read-only property
(是否就緒,只讀)
dependencies - read-only property
(從屬關係,只讀)
queuePriority - readable and writable property
(隊列中優先程度,讀寫)
completionBlock - readable and writable property
(完成後執行之block,讀寫)
Although you can attach observers to these properties, you should not use Cocoa bindings to bind them to elements of your application’s user interface. Code associated with your user interface typically must execute only in your application’s main thread. Because an operation may execute in any thread, KVO notifications associated with that operation may similarly occur in any thread.
雖然可以為這些屬性添加觀察員,但不應該將之綁定至程式的用戶介面元素上。與用戶界面相關的操作必需在主線程中執行,而與KVO通知關聯的管理器有可能在任何線程中執行。
If you provide custom implementations for any of the preceding properties, your implementations must maintain KVC and KVO compliance. If you define additional properties for your NSOperation objects, it is recommended that you make those properties KVC and KVO compliant as well. For information on how to support key-value coding, see Key-Value Coding Programming Guide. For information on how to support key-value observing, see Key-Value Observing Programming Guide.
如果欲自行實現之前的屬性,必需保持KVC及KVO。如果為工作物件自定義額外屬性,建議使其遵守KVC及KVO。如何支援鍵值編程的資訊,請見Key-Value Coding Programming Guide。如何支援鍵值觀察的資訊,請見Key-Value Observing Programming Guide。 Multicore Considerations
多核心的注意事項
The NSOperation class is itself multicore aware. It is therefore safe to call the methods of an NSOperation object from multiple threads without creating additional locks to synchronize access to the object. This behavior is necessary because an operation typically runs in a separate thread from the one that created and is monitoring it.
工作物件(NSOperation)清楚的意識到自身需要支援多核心。因此,不需要為其添加額外的鎖去同步存取物件,就可以安全的呼叫工作物件(NSOperation)的方法。這是因為其都是默認執行在不同的線程。
When you subclass NSOperation, you must make sure that any overridden methods remain safe to call from multiple threads. If you implement custom methods in your subclass, such as custom data accessors, you must also make sure those methods are thread-safe. Thus, access to any data variables in the operation must be synchronized to prevent potential data corruption. For more information about synchronization, see Threading Programming Guide.
當為工作物件(NSOperation)創建子類時,必需確定覆寫的方法在多線程中是安全的。如果在子類的自定義的實現方法中使用了檔案的存取,必需確認其為線程安全的。因此,在工作中存取任何數據變數必需使用同步的方法,以防止有可能的錯誤。更多有關同步的資訊,請見Threading Programming Guide。
Concurrent Versus Non-Concurrent Operations
並行與非並行工作物件
If you plan on executing an operation object manually, instead of adding it to a queue, you can design your operation to execute in a concurrent or non-concurrent manner. Operation objects are non-concurrent by default. In a non-concurrent operation, the operation’s task is performed synchronously—that is, the operation object does not create a separate thread on which to run the task. Thus, when you call the start method of a non-concurrent operation directly from your code, the operation executes immediately in the current thread. By the time the start method of such an object returns control to the caller, the task itself is complete.
如果想手動執行工作物件,而不是將之添加至隊列來執行,可以自行設計其為並行或非並行的工作物件。默認情況下,工作物件都是非並行的。在非並行的工作物件中,工作物件的任務都是同步執行的—也就是說,工作物件不會創建不同的線程來執行其任務。因此,當在程式碼中呼叫非並行工作物件的-(void)start方法時,將會立即在當前線程執行之。直到完成才會把控制權交回。
In contrast to a non-concurrent operation, which runs synchronously, a concurrent operation runs asynchronously. In other words, when you call the start method of a concurrent operation, that method could return before the corresponding task is completed. This might happen because the operation object created a new thread to execute the task or because the operation called an asynchronous function. It does not actually matter if the operation is ongoing when control returns to the caller, only that it could be ongoing.
相對於同步的非並行工作物件,並行工作物件是異步的。也就是說,當呼叫其-(void)start方法時,將會在完成前就將控制權交回。會這樣是因為其創建了新的線程來為其執行工作,或者其使用了異步的函數。工作物件在控制權傳回時執行其任務,這是沒有關係的,因為就該這麼做。
If you always plan to use queues to execute your operations, it is simpler to define them as non-concurrent. If you execute operations manually, though, you might want to define your operation objects as concurrent to ensure that they always execute asynchronously. Defining a concurrent operation requires more work, because you have to monitor the ongoing state of your task and report changes in that state using KVO notifications. But defining concurrent operations can be useful in cases where you want to ensure that a manually executed operation does not block the calling thread.
如果使用隊列來執行工作物件,就將之設定為非並行即可。如果是手動執行,可能需要使用並行來確保其可以異步的執行。宣告並行的工作物件會造成更多的負擔,因為必需自行監控其執行的狀態以及使用KVO通知變更項目。但宣告並行工作物件可以在特定情況下更好發揮,像是需要確認手動執行的工作物件不會阻塞該線程的時候。
For information on how to define both concurrent and non-concurrent operations, see the subclassing notes.
更多有關如何定義並行及非並行的工作物件,請見subclassing notes。
Note: In OS X v10.6, operation queues ignore the value returned by isConcurrent and always call the start method of your operation from a separate thread. In OS X v10.5, however, operation queues create a thread only if isConcurrent returns NO. In general, if you are always using operations with an operation queue, there is no reason to make them concurrent.
備註:在OS X v10.6的版本中,隊列管理器會忽略工作物件的-(BOOL)isConcurrent的傳回值,並在不同線程中執行其-(void)start方法。而在OS X v10.5版本中,管理器會在其-(BOOL)isConcurrent傳回NO時創建新的線程。事實上,如果使用隊列管理器來控制工作物件的話,沒有理由使其並行。
Subclassing Notes
子類化備註
The NSOperation class provides the basic logic to track the execution state of your operation but otherwise must be subclassed to do any real work. How you create your subclass depends on whether your operation is designed to execute concurrently or non-concurrently.
工作物件(NSOperation)會提供基本邏輯去追蹤其執行的狀態,但其餘實際的任務必需自行設定。如何創建自己子類是決定於工作物件欲為並行還是非並行的。
Methods to Override
需覆寫的方法
For non-concurrent operations, you typically override only one method:
非並行的工作物件,通常只需覆寫一個方法:
main
Into this method, you place the code needed to perform the given task. Of course, you should also define a custom initialization method to make it easier to create instances of your custom class. You might also want to define getter and setter methods to access the data from the operation. However, if you do define custom getter and setter methods, you must make sure those methods can be called safely from multiple threads.
將需要執行的任務置入此方法之中。當然,也應該自定義一個初始化的方法,來使創建工作物件更為簡單。也許也需要定義一些存取器(getter, setter)來從工作物件存取數據。但如果定義了存取器,就必需確認其操作在多線程中是安全的。
If you are creating a concurrent operation, you need to override the following methods at a minimum:
如果要創建並行的工作物件,至少要覆寫以下方法:
start
isConcurrent
isExecuting
isFinished
In a concurrent operation, your start method is responsible for starting the operation in an asynchronous manner. Whether you spawn a thread or call an asynchronous function, you do it from this method. Upon starting the operation, your start method should also update the execution state of the operation as reported by the isExecuting method. You do this by sending out KVO notifications for the isExecuting key path, which lets interested clients know that the operation is now running. Your isExecuting method must also return the status in a thread-safe manner.
在並行的工作物件中,其-(void)start方法將需要以異步的方式負責開始執行此工作。創建線程或者使用異步函數,皆需要由此方法執行。在將要開始執行時,其-(void)start方法需要更新其執行狀態,交由-(BOOL)isExecuting方法回報。也就是需要為鍵值isExecuting發送KVO通知,讓觀察其鍵值的觀察者得知目前此工作物件正在執行中。其-(BOOL)isExecuting方法也必需以線程安全的方式傳回目前的狀態。
Upon completion or cancellation of its task, your concurrent operation object must generate KVO notifications for both the isExecuting and isFinished key paths to mark the final change of state for your operation. (In the case of cancellation, it is still important to update the isFinished key path, even if the operation did not completely finish its task. Queued operations must report that they are finished before they can be removed from a queue.) In addition to generating KVO notifications, your overrides of the isExecuting and isFinished methods should also continue to return accurate values based on the state of your operation.
在工作將完成或者取消時,並行的工作物件必需為鍵值isExecuting及isFinished發送KVO通知,為工作物件標記其最後狀態。(在取消的狀況下,更新其isFinished鍵值還是很重要的,就算工作沒執行完亦是如此,隊列管理器只有在其完成的狀態下才會執行移除動作。)除了發送KVO通知之外,還必需覆寫其-(BOOL)isExecuting和-(BOOL)isFinished方法來傳回準確的狀態。
For additional information and guidance on how to define concurrent operations, see Concurrency Programming Guide.
定義並行工作物件的額外資訊及指南,請見Concurrency Programming Guide。
Important At no time in your start method should you ever call super. When you define a concurrent operation, you take it upon yourself to provide the same behavior that the default start method provides, which includes starting the task and generating the appropriate KVO notifications. Your start method should also check to see if the operation itself was cancelled before actually starting the task. For more information about cancellation semantics, see “Responding to the Cancel Command.”
在-(void)start方法中,絕對不要呼叫父類。定義並行工作物件時,需要自行提供與其默認行為相同的方法,包括開始執行工作及適當的KVO通知。-(void)start方法也需要檢查其是否在開始前就已經被取消了。更多有關取消所代表意思的資訊,請見Responding to the Cancel Command。
Even for concurrent operations, there should be little need to override methods other than those described above. However, if you customize the dependency features of operations, you might have to override additional methods and provide additional KVO notifications. In the case of dependencies, this would likely only require providing notifications for the isReady key path. Because the dependencies property is used to manage the list of dependent operations, changes to it are already handled by the default NSOperation class.
甚至,某些並行工作物件還需要覆寫前述以外的方法。如果自定義了從屬關係的部分,就必需覆寫額外的方法來提供其KVO通知,也就是需要提供其鍵值isReady的KVO通知。因為dependencies屬性是用來管理其依賴的工作物件列表的,而該屬性默認是由的父類(NSOperation)來操作變更。
Maintaining Operation Object States
持續更新工作物件當前的狀態
Operation objects maintain state information internally to determine when it is safe to execute and also to notify external clients of the progression through the operation’s life cycle. Your custom subclasses must maintain this state information to ensure the correct execution of operations in your code. Table 1 lists the key paths associated with an operation’s states and how you should manage that key path in any custom subclasses.
工作物件會持續更新其狀態訊息來決定確認其是否可安全執行及通知外部使用者其生命週期。子類化的工作物件必需包含這些資訊來保證其在程式碼中正確執行。Table 1表示了其鍵值與工作狀態的關係,還有如何在子類中管理這些鍵值的方法。
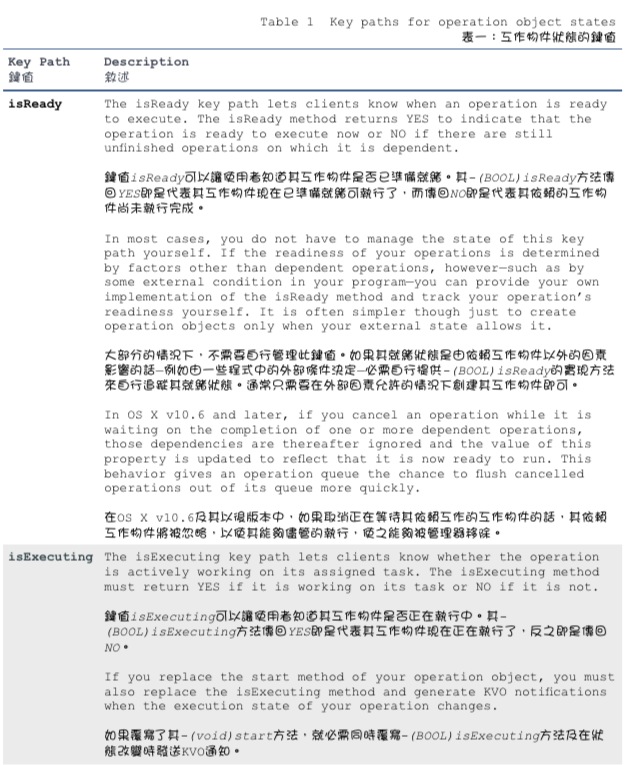
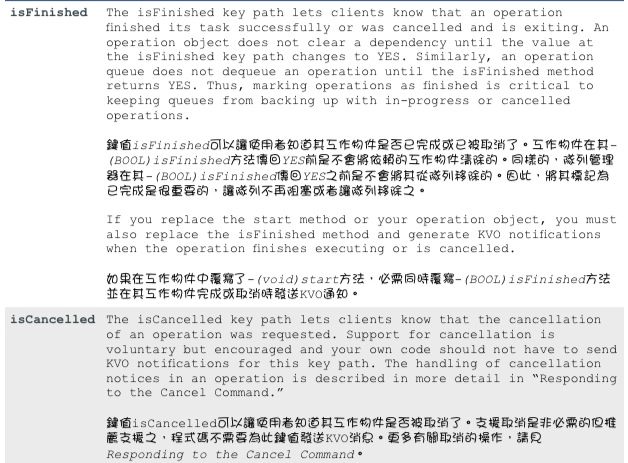
Responding to the Cancel Command
為取消事件發送訊息
Once you add an operation to a queue, the operation is out of your hands. The queue takes over and handles the scheduling of that task. However, if you decide later that you do not want to execute the operation after all—because the user pressed a cancel button in a progress panel or quit the application, for example—you can cancel the operation to prevent it from consuming CPU time needlessly. You do this by calling the cancel method of the operation object itself or by calling the cancelAllOperations method of the NSOperationQueue class.
每當添加工作物件至隊列,該物件即交由管理器控制,管理器將接收其控制權為其排程。其後如果不想讓之執行—因為用戶按下取消按鈕或者離開了程式—可以將其工作物件取消,使其不會佔有CPU。取消的方法有兩種,呼叫該工作物件的-(void)cancel方法,或者呼叫其管理器(NSOperationQueue)的-(void)cancelAllOperations方法。
Canceling an operation does not immediately force it to stop what it is doing. Although respecting the value returned by the isCancelled is expected of all operations, your code must explicitly check the value returned by this method and abort as needed. The default implementation of NSOperation does include checks for cancellation. For example, if you cancel an operation before its start method is called, the start method exits without starting the task.
取消工作物件不會立刻的使其立即停止。因工作物件必需為其的-(BOOL)isCancelled方法負責,因為此方法會影響到所有與之有關的工作物件,所以,程式碼必需檢查此方法的傳回值來確認。默認工作物件(NSOperation)的實現方法有包括了其取消方法。如果在工作物件的-(void)start方法呼叫前即將其取消,該-(void)start方法會直接結束,不執行其任務部分。
Note: In OS X v10.6, the behavior of the cancel method varies depending on whether the operation is currently in an operation queue. For unqueued operations, this method marks the operation as finished immediately, generating the appropriate KVO notifications. For queued operations, it simply marks the operation as ready to execute and lets the queue call its start method, which subsequently exits and results in the clearing of the operation from the queue.
備註:在OS X v10.6的版本中,取消的行為是取決於其是否當前存在隊列中。對非隊列中的工作物件來說,此方法會立即將自身標記為已完成,並發送其KVO通知。對於隊列中的工作物件來說,會將其標記為就緒的狀態使其管理器執行其-(void)start方法來檢查,使之能夠隨後立即結束並從隊列中移除。
You should always support cancellation semantics in any custom code you write. In particular, your main task code should periodically check the value of the isCancelled method. If the method ever returns YES, your operation object should clean up and exit as quickly as possible. If you implement a custom start method, that method should include early checks for cancellation and behave appropriately. Your custom start method must be prepared to handle this type of early cancellation.
在任何自定義的工作物件中,應該都要支援其取消狀態。尤其是在主要的方法中,應該要經常檢查-(BOOL)isCancelled方法傳回的值。一旦其值傳回YES,必需將當前工作立刻清除使之能夠盡快的離開。如果自行實現了-(void)start方法,該方法必需包含了前置檢查其取消狀態及其適當的行為。
In addition to simply exiting when an operation is cancelled, it is also important that you move a cancelled operation to the appropriate final state. Specifically, if you manage the values for the isFinished and isExecuting properties yourself (perhaps because you are implementing a concurrent operation), you must update those variables accordingly. Specifically, you must change the value returned by isFinished to YES and the value returned by isExecuting to NO. You must make these changes even if the operation was cancelled before it started executing.
除了在其取消狀態下要結束離開之外,同時也要將該工作物件設定至最終狀態。尤其是自行管理isFinished及isExecuting屬性的人(或許是因為實現並行工作物件),必需根據其值更新這些變數值,將其-(BOOL)isFinished方法傳回的值改為YES,以及將其-(BOOL)isExecuting方法傳回的值改為NO。甚至是在其開始執行前被取消亦需如此。
Tasks
方法
Initialization
初始化
– init
Executing the Operation
執行工作
– start
– main
– completionBlock
– setCompletionBlock:
Canceling Operations
取消工作
– cancel
Getting the Operation Status
取得狀態
– isCancelled
– isExecuting
– isFinished
– isConcurrent
– isReady
Managing Dependencies
管理依賴關係
– addDependency:
– removeDependency:
– dependencies
Prioritizing Operations in an Operation Queue
隊列中的優先程度
– queuePriority
– setQueuePriority:
Managing the Execution Priority
管理執行優先順序
– threadPriority
– setThreadPriority:
Waiting for Completion
等待執行
– waitUntilFinished Instance Methods
實例方法
addDependency:
Makes the receiver dependent on the completion of the specified operation.
使當前工作物件依賴於特定的工作物件。
- (void)addDependency:(NSOperation *)operation
Parameters
參數
operation
The operation on which the receiver should depend. The same dependency should not be added more than once to the receiver, and the results of doing so are undefined.
欲要被依賴的工作物件。同樣的工作物件不可以重復添加依賴至當前工作物件,否則會造成無法預期的後果。
Discussion
討論
The receiver is not considered ready to execute until all of its dependent operations have finished executing. If the receiver is already executing its task, adding dependencies has no practical effect. This method may change the isReady and dependencies properties of the receiver.
當前物件在其依賴工作物件執行完成前是不會執行的。如此欲依賴的工作物件在其被執行完成後才添加至當前工作物件,是沒有效果的。此方法會影響當前工作物件的isReady和dependencies屬性。
It is a programmer error to create any circular dependencies among a set of operations. Doing so can cause a deadlock among the operations and may freeze your program.
循環依賴可能會導致程式崩潰,編程者應避免此情況發生。
Availability
支援
Available in iOS 2.0 and later.
支援iOS 2.0及其以後的版本。
See Also
參見
– removeDependency:
– dependencies
Declared In
宣告於
NSOperation.h
cancel
Advises the operation object that it should stop executing its task.
通知當前工作物件取消執行工作。
- (void)cancel
Discussion
討論
This method does not force your operation code to stop. Instead, it updates the object’s internal flags to reflect the change in state. If the operation has already finished executing, this method has no effect. Canceling an operation that is currently in an operation queue, but not yet executing, makes it possible to remove the operation from the queue sooner than usual.
此方法並不會將工作物件的程式碼停止。取而代之的是會更新其標記,反應狀態的變更。如此當前工作物件已執行完成,將不會有任何效果。如果是取消在隊列中尚未執行的工作物件,此方法會以最快的速度將此工作物件從隊列中移除。
In OS X v10.6 and later, if an operation is in a queue but waiting on unfinished dependent operations, those operations are subsequently ignored. Because it is already cancelled, this behavior allows the operation queue to call the operation’s start method sooner and clear the object out of the queue. If you cancel an operation that is not in a queue, this method immediately marks the object as finished. In each case, marking the object as ready or finished results in the generation of the appropriate KVO notifications.
在OS X v10.6及其以後的版本中,如果當前物件正在隊列中等待其依賴的工作物件時,那些工作物件將會被忽略,因為當前物件被取消,所以這樣的行為可以讓管理器更快的呼叫其-(void)start方法,從而使之被從隊列中移除。如果當前工作物件並不在隊列當中,此方法將會使當前物件立即標記為完成。在此兩種情況中,將工作物件標記為就緒或已完成都將會一系列的KVO通知。
In versions of OS X prior to 10.6, an operation object remains in the queue until all of its dependencies are removed through the normal processes. Thus, the operation must wait until all of its dependent operations finish executing or are themselves cancelled and have their start method called.
在OS X v10.6之前的版本中,當前工作物件將會保持在隊列中直到其依賴工作物件正常執行完。因此,當前工作物件將會等到其依賴工作物件完成或取消後才會呼叫當前工作物件的-(void)start方法。
For more information on what you must do in your operation objects to support cancellation, see “Responding to the Cancel Command.”
更多有關讓工作物件支援取消的訊息,請見Responding to the Cancel Command。
Availability
支援
Available in iOS 2.0 and later.
支援iOS 2.0及其以後的版本。
See Also
參見
– isCancelled
Declared In
宣告於
NSOperation.h
completionBlock
Returns the block to execute when the operation’s main task is complete.
傳回當前工作物件的-(void)main方法執行完成後會執行的block物件。
- (void (^)(void))completionBlock
Return Value
傳回值
The block to execute after the operation’s main task is completed. This block takes no parameters and has no return value.
當前工作物件的-(void)main方法執行完成後會執行的block物件。此物件沒有任何參數和傳回值。
Discussion
討論
The completion block you provide is executed when the value returned by the isFinished method changes to YES. Thus, this block is executed by the operation object after the operation’s primary task is finished or cancelled.
此block物件將會在工作物件的-(BOOL)isFinished方法傳回YES時執行。因此,此物件將有可能在其完成或取消的狀況下執行。
Availability
支援
Available in iOS 4.0 and later.
支援iOS 4.0及其以後的版本。
See Also
參見
– setCompletionBlock:
Declared In
宣告於
NSOperation.h
dependencies
Returns a new array object containing the operations on which the receiver is dependent.
傳回當前工作物件所依賴工作物件組成的新陣列。
- (NSArray *)dependencies
Return Value
傳回值
A new array object containing the NSOperation objects.
包含工作物件(NSOperation)的新陣列。
Discussion
討論
The receiver is not considered ready to execute until all of its dependent operations finish executing.
當前物件在其依賴工作物件完成前是不會就緒的。
Operations are not removed from this dependency list as they finish executing. You can therefore use this list to track all dependent operations, including those that have already finished executing. The only way to remove an operation from this list is to use the removeDependency: method.
此清單中的工作物件在其執行完成後是不會被移除的。可以透過此來追蹤其依賴的工作物件,包括那些已經執行完成的。從此清單中移除的唯一方法只是透過使用其removeDependency:方法。
Availability
支援
Available in iOS 2.0 and later.
支援iOS 2.0及其以後的版本。
See Also
參見
– addDependency:
– removeDependency:
Declared In
宣告於
NSOperation.h
init
Returns an initialized NSOperation object.
傳回初始的工作物件(NSOperation)。
- (id)init
Return Value
傳回值
The initialized NSOperation object.
初始的工作物件(NSOperation)。
Discussion
討論
Your custom subclasses must call this method. The default implementation initializes the object’s instance variables and prepares it for use.
自定義的子類必需呼叫此方法。此方法默認實現初始化物件變數,使其可以使用。
Availability
支援
Available in iOS 2.0 and later.
支援iOS 2.0及其以後的版本。
Related Sample Code
相關範例
LazyTableImages
ListAdder
SeismicXML
Declared In
宣告於
NSOperation.h
isCancelled
Returns a Boolean value indicating whether the operation has been cancelled.
傳回表示是否被取消的布林值。
- (BOOL)isCancelled
Return Value
傳回值
YES if the operation was explicitly cancelled by an invocation of the receiver’s cancel method; otherwise, NO. This method may return YES even if the operation is currently executing.
當工作物件被以-(void)cancel方法取消時,傳回YES。反之,傳回NO。甚至有可能正在執行中也會傳回YES。
Discussion
討論
Canceling an operation does not actively stop the receiver’s code from executing. An operation object is responsible for calling this method periodically and stopping itself if the method returns YES.
取消工作物件並不會將其程式碼停止。程式碼應該時常檢查此值來使其自身中止執行。
You should always call this method before doing any work towards accomplishing the operation’s task, which typically means calling it at the beginning of your custom main method. It is possible for an operation to be cancelled before it begins executing or at any time while it is executing. Therefore, calling this method at the beginning of your main method (and periodically throughout that method) lets you exit as quickly as possible when an operation is cancelled.
應該要在完成工作之前呼叫此方法,一般大概都是在自定義工作物件的-(void)main方法開頭呼叫。其有可能在執行前或執行中被取消。因此,在-(void)main方法的開頭(或經常的在各處呼叫)呼叫可以使已被取消的工作物件盡快結束離開。
Availability
支援
Available in iOS 2.0 and later.
支援iOS 2.0及其以後的版本。
See Also
參見
– cancel
Related Sample Code
相關範例
LazyTableImages
ListAdder
Declared In
宣告於
NSOperation.h
i
sConcurrent
Returns a Boolean value indicating whether the operation runs asynchronously.
傳回表示是否異步執行的布林值。
- (BOOL)isConcurrent
Return Value
傳回值
YES if the operation runs asynchronously with respect to the current thread or NO if the operation runs synchronously on whatever thread started it. This method returns NO by default.
如果在以異步執行則傳回YES,如果以同步執行則傳回NO。默認值為NO。
Discussion
討論
If you are implementing a concurrent operation, you must override this method and return YES from your implementation. For more information about the differences between concurrent and non-concurrent operations, see “Concurrent Versus Non-Concurrent Operations.”
如果欲實現並行的工作物件,必需覆寫此方法並使之傳回YES。更多有關並行與非並行的差異,請見Concurrent Versus Non-Concurrent Operations。
In OS X v10.6 and later, operation queues ignore the value returned by this method and always start operations on a separate thread.
在OS X v10.6及其以後的版本中,管理器將會忽略此方法之傳回值,並將工作物件執行於不同的線程中。
Availability
支援
Available in iOS 2.0 and later.
支援iOS 2.0及其以後的版本。
Declared In
宣告於
NSOperation.h
isExecuting
Returns a Boolean value indicating whether the operation is currently executing.
傳回表示當前工作物件是否正在執行的布林值。
- (BOOL)isExecuting
Return Value
傳回值
YES if the operation is executing; otherwise, NO if the operation has not been started or is already finished.
正在執行則傳回YES。還沒執行或者完成執行則傳回NO。
Discussion
討論
If you are implementing a concurrent operation, you should override this method to return the execution state of your operation. If you do override it, be sure to generate KVO notifications for the isExecuting key path whenever the execution state of your operation object changes. For more information about manually generating KVO notifications, see Key-Value Observing Programming Guide.
如果實現了並行工作物件,必需要覆寫此方法來傳回當前工作物件的狀態,並確保在其isExecuting鍵值改變時為其發送KVO通知。更多有關手動發送KVO通知的訊息,請見Key-Value Observing Programming Guide。
Availability
支援
Available in iOS 2.0 and later.
支援iOS 2.0及其以後的版本。
Declared In
宣告於
NSOperation.h
isFinished
Returns a Boolean value indicating whether the operation is done executing.
傳回表示是否完成執行的布林值。
- (BOOL)isFinished
Return Value
YES if the operation is no longer executing; otherwise, NO.
如果不再執行則傳回YES,否則傳回NO。
Discussion
If you are implementing a concurrent operation, you should override this method and return a Boolean to indicate whether your operation is currently finished. If you do override it, be sure to generate appropriate KVO notifications for the isFinished key path when the completion state of your operation object changes. For more information about manually generating KVO notifications, see Key-Value Observing Programming Guide.
如果實現了並行工作物件,必需要覆寫此方法,使其在完成時能夠傳回正確的布林值,並確保在其isFinished鍵值改變時為其發送KVO通知。更多有關手動發送KVO通知的訊息,請見Key-Value Observing Programming Guide。
Availability
支援
Available in iOS 2.0 and later.
支援iOS 2.0及其以後的版本。
Declared In
宣告於
NSOperation.h
isReady
Returns a Boolean value indicating whether the receiver’s operation can be performed now.
傳回表示當前工作物件是否準備就緒的布林值。
- (BOOL)isReady
Return Value
傳回值
YES if the operation can be performed now; otherwise, NO.
如果準備就緒則傳回YES,否則傳回NO。
Discussion
Operations may not be ready due to dependencies on other operations or because of external conditions that might prevent needed data from being ready. The NSOperation class manages dependencies on other operations and reports the readiness of the receiver based on those dependencies.
未就緒的原因有可能是其依賴工作物件或者外部條件所致。工作物件(NSOperation)會管理其依賴關係並基於其依賴對象回報其就緒狀態。
If you want to use custom conditions to determine the readiness of your operation object, you can override this method and return a value that accurately reflects the readiness of the receiver. If you do so, your custom implementation should invoke super and incorporate its return value into the readiness state of the object. Your custom implementation must also generate appropriate KVO notifications for the isReady key path.
如果欲使用自定義的條件來決定工作物件的就緒狀態,必需覆寫此方法以傳回準確的狀態。若是這麼做的話,在方法中必需要呼叫其父類將其就緒狀態與欲傳回值合併。自定義的實現必需包含其isReady鍵值的KVO通知。
Availability
支援
Available in iOS 2.0 and later.
支援iOS 2.0及其以後的版本。
See Also
參見
– dependencies
Declared In
宣告於
NSOperation.h
main
Performs the receiver’s non-concurrent task.
執行工作物件的非並行任務。
- (void)main
Discussion
討論
The default implementation of this method does nothing. You should override this method to perform the desired task. In your implementation, do not invoke super.
默認的此方法是空的。必需自行覆寫此方法來執行任務。在實現中,不可以呼叫其父類。
If you are implementing a concurrent operation, you are not required to override this method but may do so if you plan to call it from your custom start method.
如果實現的是並行工作物件,不需要覆寫此方法,但也可以為了可以從-(void)start方法中呼叫而覆寫。
Availability
支援
Available in iOS 2.0 and later.
支援iOS 2.0及其以後的版本。
See Also
參見
– start
Declared In
宣告於
NSOperation.h
queuePriority
Returns the priority of the operation in an operation queue.
傳回於隊列中的優先程度。
- (NSOperationQueuePriority)queuePriority
Return Value
傳回值。
The relative priority of the operation. The returned value always corresponds to one of the predefined constants. (For a list of valid values, see “Operation Priorities.”) If no priority is explicitly set, this method returns NSOperationQueuePriorityNormal.
工作物件相關的優先程度。此值將會指向已定義過的常量。(實際的值,請見Operation Priorities。)如果未指定,默認傳回NSOperationQueuePriorityNormal。
Availability
支援
Available in iOS 2.0 and later.
支援iOS 2.0及其以後的版本。
See Also
參見
– setQueuePriority:
Declared In
宣告於
NSOperation.h
removeDependency:
Removes the receiver’s dependence on the specified operation.
移除工作物件的依賴關係。
- (void)removeDependency:(NSOperation *)operation
Parameters
參數
operation
The dependent operation to be removed from the receiver.
要被移除依賴關係的工作物件。
Discussion
討論
This method may change the isReady and dependencies properties of the receiver.
此方法會影響工作物件的isReady與dependencies屬性。
Availability
支援
Available in iOS 2.0 and later.
支援iOS 2.0及其以後的版本。
See Also
參見
– addDependency:
– dependencies
Declared In
宣告於
NSOperation.h
setCompletionBlock:
Sets the block to execute when the operation has finished executing.
設定工作完成後將會執行的block物件。
- (void)setCompletionBlock:(void (^)(void))block
Parameters
參數
block
The block to be executed when the operation finishes. This method creates a copy of the specified block. The block itself should take no parameters and have no return value.
工作完成後將會執行的block物件。此方法會以複製的形式將block傳入。此block沒有參數及傳回值。
Discussion
討論
The exact execution context for your completion block is not guaranteed but is typically a secondary thread. Therefore, you should not use this block to do any work that requires a very specific execution context. Instead, you should shunt that work to your application’s main thread or to the specific thread that is capable of doing it. For example, if you have a custom thread for coordinating the completion of the operation, you could use the completion block to ping that thread.
此block的執行環境不能保證,但通常是在次要線程。因此,不應該使用此物件來執行需要於特定環境執行的任務。取而代之的是,將該任務轉入主線程或者能夠勝任的特定線程。如果擁有一個自定義用來協議工作物件實現的線程,也可以將block送入其中。
Because the completion block executes after the operation indicates it has finished its task, you must not use a completion block to queue additional work considered to be part of that task. An operation object whose isFinished method returns YES must be done with all of its task-related work by definition. The completion block should be used to notify interested objects that the work is complete or perform other tasks that might be related to, but not part of, the operation’s actual task.
因為此block將會於工作物件完成後才執行,所以不可以使用其來隊列其自身的部分工作。工作物件所定義的工作完成後,其-(BOOL)isFinished方法才會傳回YES。此block應該用於通知與此工作物件有關聯的物件,而不是用於執行此工作物件的部分內容。
A finished operation may finish either because it was cancelled or because it successfully completed its task. You should take that fact into account when writing your block code. Similarly, you should not make any assumptions about the successful completion of dependent operations, which may themselves have been cancelled.
已完成的工作物件有可能是被取消了或是成功的完成了任務。創建block時,應該要將各種情況考慮進去,同時不應該任意的認定其是被取消了亦或是成功的執行。
Availability
支援
Available in iOS 4.0 and later.
支援iOS 4.0及其以後的版本。
Declared In
宣告於
NSOperation.h
setQueuePriority:
Sets the priority of the operation when used in an operation queue.
設定工作物件於隊列中的優先程度。
- (void)setQueuePriority:(NSOperationQueuePriority)priority
Parameters
參數
priority
The relative priority of the operation. For a list of valid values, see “Operation Priorities.”
與工作物件關聯的優先程度。實際的值,請見Operation Priorities。
Discussion
討論
You should use priority values only as needed to classify the relative priority of non-dependent operations. Priority values should not be used to implement dependency management among different operation objects. If you need to establish dependencies between operations, use the addDependency: method instead.
此優先程度只應該使用於非並行的工作物件。優先程度不應該使用於實現不同工作物件間的依賴關係。如果需要設定工作物件間的依賴關係,請使用addDependency:方法。
If you attempt to specify a priority value that does not match one of the defined constants, this method automatically adjusts the value you specify towards the NSOperationQueuePriorityNormal priority, stopping at the first valid constant value. For example, if you specified the value -10, this method would adjust that value to match the NSOperationQueuePriorityVeryLow constant. Similarly, if you specified +10, this method would adjust the value to match the NSOperationQueuePriorityVeryHigh constant.
如果試圖以定義常量以外的值來創建優先程度,此方法將會自動的將值往NSOperationQueuePriorityNormal的方向調整,以最近的值設定之。比如,如果指定值-10,此方法將自動調整為NSOperationQueuePriorityVeryLow。同樣的,如果指定值+10,將調整為NSOperationQueuePriorityVeryHigh。
Availability
支援
Available in iOS 2.0 and later.
支援iOS 2.0及其以後的版本。
See Also
參見
– queuePriority
– addDependency:
Declared In
宣告於
NSOperation.h
setThreadPriority:
Sets the thread priority to use when executing the operation.
設定執行工作物件時的線程優先程度。
- (void)setThreadPriority:(double)priority
Parameters
參數
priority
The new thread priority, specified as a floating-point number in the range 0.0 to 1.0, where 1.0 is the highest priority.
新的線程優先程度值,指定0.0至1.0的浮點數,1.0為最優先值。
Discussion
討論
The value you specify is mapped to the operating system’s priority values. The specified thread priority is applied to the thread only while the operation’s main method is executing. It is not applied while the operation’s completion block is executing. For a concurrent operation in which you create your own thread, you must set the thread priority yourself in your custom start method and reset the original priority when the operation is finished.
指定的值為系統的優先程度值。該線程優先度只對執行其-(void)main方法時有效,對其completionBlock無效。對於在自行創建的線程中執行的並行工作物件來說,必需在自定義的-(void)start方法中設定其線程優先程度,並於工作物件完成時重置其值。
Availability
支援
Available in iOS 4.0 and later.
支援iOS 4.0及其以後的版本。
See Also
參見
setThreadPriority: (NSThread)
Declared In
宣告於
NSOperation.h
start
Begins the execution of the operation.
開始工作物件的執行。
- (void)start
Discussion
討論
The default implementation of this method updates the execution state of the operation and calls the receiver’s main method. This method also performs several checks to ensure that the operation can actually run. For example, if the receiver was cancelled or is already finished, this method simply returns without calling main. (In OS X v10.5, this method throws an exception if the operation is already finished.) If the operation is currently executing or is not ready to execute, this method throws an NSInvalidArgumentException exception. In OS X v10.5, this method catches and ignores any exceptions thrown by your main method automatically. In OS X v10.6 and later, exceptions are allowed to propagate beyond this method. You should never allow exceptions to propagate out of your main method.
此方法默認為此工作物件實現更新當前執行狀態以及呼叫其-(void)main方法。此方法會多次檢查其狀態來確保工作物件能順利執行。若是工作物件已取消或是已完成,此方法將會直接結束離開而不呼叫-(void)main方法。(在OS X v10.5的版本中,若是執行時工作物件已完成了的話,將會拋出異常。)如果工作物件正在執行或者還沒準備就緒,將會拋出NSInvalidArgumentException異常。在OS X v10.5的版本中,此方法將會自動將-(void)main方法中所有拋出的異常攔截並忽略掉。在OS X v10.6及其以後的版本中,異常不允許超出此方法。自定義時亦不該使異常超出-(void)main方法。
Note: An operation is not considered ready to execute if it is still dependent on other operations that have not yet finished.
備註:工作物件在其依賴的工作物件執行完成前是不會執行的。
If you are implementing a concurrent operation, you must override this method and use it to initiate your operation. Your custom implementation must not call super at any time. In addition to configuring the execution environment for your task, your implementation of this method must also track the state of the operation and provide appropriate state transitions. When the operation executes and subsequently finishes its work, it should generate KVO notifications for the isExecuting and isFinished key paths respectively. For more information about manually generating KVO notifications, see Key-Value Observing Programming Guide.
如果實現了並行的工作物件,必需要覆寫此方法並使用其來初始化工作物件,並且不允許呼叫其父類。除了為之設定其執行環境之外,此方法的實現也必需追蹤工作物件的狀態來為其提供狀態資訊。當完成工作時,應該要負責任的為其isExecuting和isFinished鍵值發送KVO通知。更多有關手動實現KVO通知的資訊,請見Key-Value Observing Programming Guide。
You can call this method explicitly if you want to execute your operations manually. However, it is a programmer error to call this method on an operation object that is already in an operation queue or to queue the operation after calling this method. Once you add an operation object to a queue, the queue assumes all responsibility for it.
欲手動執行工作物件時,亦可以呼叫此方法。然而,若是將那些已經在隊列中或者會在此方法之後加入隊列的工作物件之此方法執行的話,就是編程者的錯誤了。一旦將工作物件加入隊列,管理器將會全權接管該物件。
Availability
支援
Available in iOS 2.0 and later.
支援iOS 2.0及其以後的版本。
See Also
參見
– main
– isReady
– dependencies
Declared In
宣告於
NSOperation.h
threadPriority
Returns the thread priority to use when executing the operation.
傳回工作物件執行時的線程優先程度。
- (double)threadPriority
Return Value
傳回值
A floating-point number in the range 0.0 to 1.0, where 1.0 is the highest priority. The default thread priority is 0.5.
從0.0至1.0的浮點數,1.0為最優先值。默認值為0.5。
Availability
支援
Available in iOS 4.0 and later.
支援iOS 4.0及其以後的版本。
See Also
參見
threadPriority
Declared In
宣告於
NSOperation.h
waitUntilFinished
Blocks execution of the current thread until the receiver finishes.
將當前線程阻塞直到工作物件完成為止。
- (void)waitUntilFinished
Discussion
討論
The receiver should never call this method on itself and should avoid calling it on any operations submitted to the same operation queue as itself. Doing so can cause the operation to deadlock. It is generally safe to call this method on an operation that is in a different operation queue, although it is still possible to create deadlocks if each operation waits on the other.
工作物件不應該自行呼叫此方法,應該避免在任何被發送至隊列的工作物件上自行呼叫此方法,否則會造成工作物件的死鎖。通常安全的使用方式應該是由不同管理器的工作物件來發送,雖然如果工作物件之間互相等待還是有可能造成死鎖。
A typical use for this method would be to call it from the code that created the operation in the first place. After submitting the operation to a queue, you would call this method to wait until that operation finished executing.
使用此方法的典型例子是由最先創建的工作物件來呼叫此方法。將工作物件發送至隊列後,可以呼叫此方法來等待直到該工作完成。
Availability
支援
Available in iOS 4.0 and later.
支援iOS 4.0及其以後的版本。
Declared In
宣告於
NSOperation.h
Constants
常量
NSOperationQueuePriority
隊列中工作優先程度
Describes the priority of an operation relative to other operations in an operation queue.
描述工作物件在隊列中的優先程度。
typedef NSInteger NSOperationQueuePriority;
Discussion
討論
For a list of related constants, see “Operation Priorities”.
相關常量的清單,請見Operation Priorities。
Availability
支援
Available in iOS 2.0 and later.
支援iOS 2.0及其以後的版本。
Declared In
宣告於
NSOperation.h
Operation Priorities
工作物件的優先程度
These constants let you prioritize the order in which operations execute.
此常量可以排列工作物件執行的優先順序。
enum {
NSOperationQueuePriorityVeryLow = -8,
NSOperationQueuePriorityLow = -4,
NSOperationQueuePriorityNormal = 0,
NSOperationQueuePriorityHigh = 4,
NSOperationQueuePriorityVeryHigh = 8
};
Constants
常量
NSOperationQueuePriorityVeryLow
Operations receive very low priority for execution.
工作執行優先程度:非常低
Available in iOS 2.0 and later.
支援iOS 2.0及其以後的版本。
Declared in NSOperation.h.
宣告於NSOperation.h
NSOperationQueuePriorityLow
Operations receive low priority for execution.
工作執行優先程度:低
Available in iOS 2.0 and later.
支援iOS 2.0及其以後的版本。
Declared in NSOperation.h.
宣告於NSOperation.h
NSOperationQueuePriorityNormal
Operations receive the normal priority for execution.
工作執行優先程度:普通
Available in iOS 2.0 and later.
支援iOS 2.0及其以後的版本。
Declared in NSOperation.h.
宣告於NSOperation.h
NSOperationQueuePriorityHigh
Operations receive high priority for execution.
工作執行優先程度:高
Available in iOS 2.0 and later.
支援iOS 2.0及其以後的版本。
Declared in NSOperation.h.
宣告於NSOperation.h
NSOperationQueuePriorityVeryHigh
Operations receive very high priority for execution.
工作執行優先程度:非常高
Available in iOS 2.0 and later.
支援iOS 2.0及其以後的版本。
Declared in NSOperation.h.
宣告於NSOperation.h
Discussion
討論
You can use these constants to specify the relative ordering of operations that are waiting to be started in an operation queue. You should always use these constants (and not the defined value) for determining priority.
可以使用這些常量來指定管理器開始執行的相關優先程度。應該使用這些定義過的常量(而不是其實際的值)來決定優先程度。
Declared In
宣告於
NSOperation.h
-END-