数据面板
效果
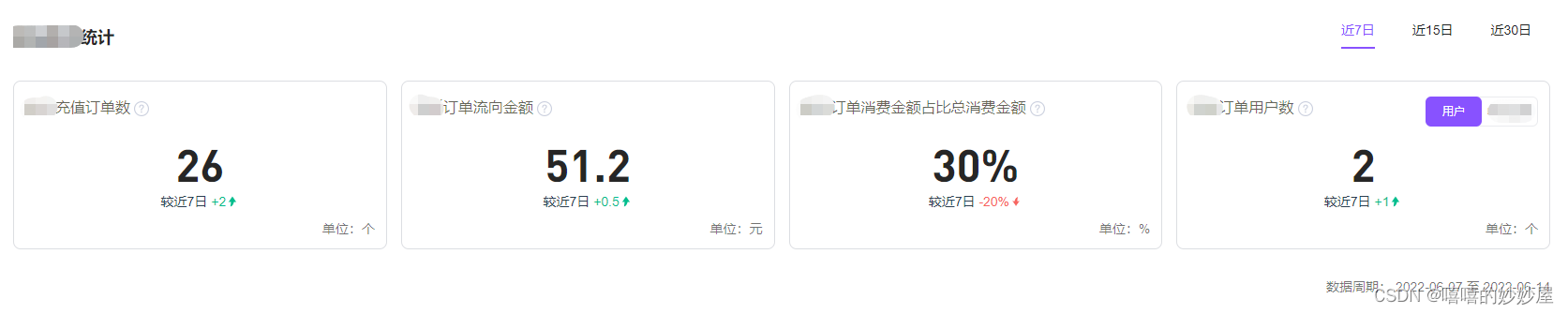
index.vue
<template>
<div class="container">
<div class="card">
<div class="header">
<div class="title">订单统计</div>
<div class="detail">
<el-tabs
class="mid"
v-model="currentDate"
@tab-click="dateGroupChange"
>
<el-tab-pane
v-for="item in Object.keys(dateMap)"
:key="item"
:label="dateMap[item]"
:name="item"
></el-tab-pane>
</el-tabs>
</div>
</div>
<div class="content">
<div class="list">
<div class="item">
<div class="text1">
充值订单数
<el-tooltip
class="tooltip"
effect="dark"
content="周期范围内有流向的订单数"
placement="right"
>
<i class="icon-question"></i>
</el-tooltip>
</div>
<p class="text2">
{{ orderCount }}
</p>
<CompareText
:result="2"
:unit="''"
:target="dateMap[currentDate]"
/>
<p class="unit">单位:个</p>
</div>
<div class="item">
<div class="text1">
订单流向金额
<el-tooltip
class="tooltip"
effect="dark"
content="周期范围内有订单流向的消费金额"
placement="right"
>
<i class="icon-question"></i>
</el-tooltip>
</div>
<p class="text2">
{{ flowCount }}
</p>
<CompareText
:result="0.5"
:unit="''"
:target="dateMap[currentDate]"
/>
<p class="unit">单位:元</p>
</div>
<div class="item">
<div class="text1">
订单消费金额占比总消费金额
<el-tooltip
class="tooltip"
effect="dark"
content="周期范围内有订单流向的消费金额在总消费金额的占比"
placement="right"
>
<i class="icon-question"></i>
</el-tooltip>
</div>
<p class="text2">
{{ proportion + '%'}}
</p>
<CompareText
:result="-20"
:unit="'%'"
:target="dateMap[currentDate]"
/>
<p class="unit">单位:%</p>
</div>
<div class="item">
<div class="title">
<div class="text1">
{{userCountTitle[userCountIndex]}}
<el-tooltip
class="tooltip"
effect="dark"
:content="userType === 'user' ? '订单用户数:周期范围内有订单的用户数' : '订单管理员:周期范围内有订单消费在店中的店家数'"
placement="right"
>
<i class="icon-question"></i>
</el-tooltip>
</div>
<div class="selectOption">
<div :class="userType === 'user' ? 'user active' : 'user'" @click="changeUserType('user')">用户</div>
<div :class="userType === 'manager' ? 'manager active' : 'manager'" @click="changeUserType('manager')">管理员</div>
</div>
</div>
<p class="text2">
{{ userCount }}
</p>
<CompareText
:result="1"
:unit="''"
:target="dateMap[currentDate]"
/>
<p class="unit">单位:个</p>
</div>
</div>
</div>
<div class="footer">
数据周期:
{{ startTime }}
至
{{ endTime }}
</div>
</div>
</div>
</template>
<style lang="scss" src="./index.scss" scoped></style>
<script src="./index.js"></script>
index.js
import CompareText from '../compareText/index.vue'
const dateMap = {
week: '近7日',
halfMonth: '近15日',
wholeMonth: '近30日'
}
const userCountTitle = {
0: '订单用户数',
1: '订单管理员数'
}
export default {
name: 'panel',
components: {
CompareText
},
data () {
return {
dateMap: dateMap,
userCountTitle: userCountTitle,
userCountIndex: 0,
currentDate: 'week',
orderCount: 26,
flowCount: 51.2,
proportion: 30,
userCount: 2,
startTime: '2022-06-07',
endTime: '2022-06-14',
userType: 'user'
}
},
computed: {},
watch: {},
mounted () {},
methods: {
dateGroupChange (target) {
this.currentDate = target.name
},
changeUserType (val) {
this.userType = val
if (this.userType === 'manager') {
this.userCountIndex = 1
} else {
this.userCountIndex = 0
}
}
}
}
index.scss
.container {
margin-bottom: 16px;
}
.tooltip {
height: 16px;
width: 16px;
.icon-question {
margin-left: 5px;
}
}
.text1 {
font-size: 16px;
color: rgba(17, 17, 0, 0.65);
}
.text2 {
text-align: center;
font-family: $DINGfont;
margin-top: 20px;
font-size: 48px;
color: rgba(0, 0, 0, 0.85);
}
.content {
overflow: hidden;
.list {
display: flex;
justify-content: space-between;
margin-bottom: 30px;
.item {
position: relative;
flex: 1;
min-width: 118px;
height: 180px;
padding: 16px 12px;
border-radius: 8px;
border: 0.5px solid #dcdee2;
.title {
display: flex;
justify-content: space-between;
margin-bottom: -27px;
}
&:hover {
border: 0.5px solid #e8eaec;
box-shadow: 0px 6px 16px -8px rgba(136, 161, 210, 0.08),
0px 9px 28px rgba(136, 161, 210, 0.05),
0px 12px 48px 16px rgba(136, 161, 210, 0.03);
}
.unit {
margin-top: 10px;
font-size: 14px;
text-align: right;
color: rgba(0, 0, 0, 0.55);
}
}
.item + .item {
margin-left: 15px;
}
}
}
.footer {
color: rgba(0, 0, 0, 0.55);
display: flex;
justify-content: right;
}
.selectOption {
display: flex;
align-items: center;
font-size: 12px;
border: 1px solid #EAEBEE;
border-radius: 6px;
width: 120px;
height: 32px;
margin-bottom: 16px;
.user, .manager {
cursor: pointer;
border-radius: 6px;
width: 60px;
height: 32px;
text-align: center;
line-height: 32px;
color: #606266;
&:hover {
font-weight: 400;
}
}
.user {
margin-left: -1px;
}
.manager {
margin-right: -1px;
}
.active {
color: #ffffff;
font-weight: 400;
background-color: rgb(136, 82, 255);
border-color: rgb(136, 82, 255);
&:hover {
background-color: rgba(136, 82, 255, 0.8);
}
}
}
compareText/index.vue
<template>
<p v-if="result > 0" class="text3 marRight10 width150">
<span class="center">较{{target}} <span class="increase">+{{ result | localeString }}{{unit}}</span><span class="up icon"></span></span>
</p>
<p v-else-if="result < 0" class="text3 marRight10 width150">
<span class="center">较{{target}} <span class="decrease">-{{ (result * -1) | localeString }}{{unit}}</span><span class="down icon"></span></span>
</p>
<p v-else class="text3 marRight10 width150">
<span class="flatText center">较{{target}}持平</span>
</p>
</template>
<script>
export default {
props: {
result: Number,
target: String,
unit: String
}
}
</script>
<style lang='scss' scoped>
.width150{
width: 100%;
}
.center{
display: flex;
align-items: center;
}
.text3{
display: flex;
align-items: center;
justify-content: center;
}
.marRight10{
margin-right: 10px;
}
.increase{
color: #00BD8D;
}
.decrease{
color: #F76560
}
.up{
background: url('~@/img/inline/icon/up.svg');
}
.down{
background: url('~@/img/inline/icon/down.svg');
}
.icon{
display: inline-block;
width: 10px;
height: 16px;
background-size: 100% 100%;
margin: 0 2px;
}
.flat{
width: 5px;
height: 5px;
background-color: #999999;
}
.flatText{
color: #999999;
}
</style>