作者:ssslinppp
1.准备
这里我们采用Hibernate-validator来进行验证,Hibernate-validator实现了JSR-303验证框架支持注解风格的验证。首先我们要到http://hibernate.org/validator/下载需要的jar包,这里以4.3.1.Final作为演示,解压后把hibernate-validator-4.3.1.Final.jar、jboss-logging-3.1.0.jar、validation-api-1.0.0.GA.jar这三个包添加到项目中。
2. Spring MVC上下文配置
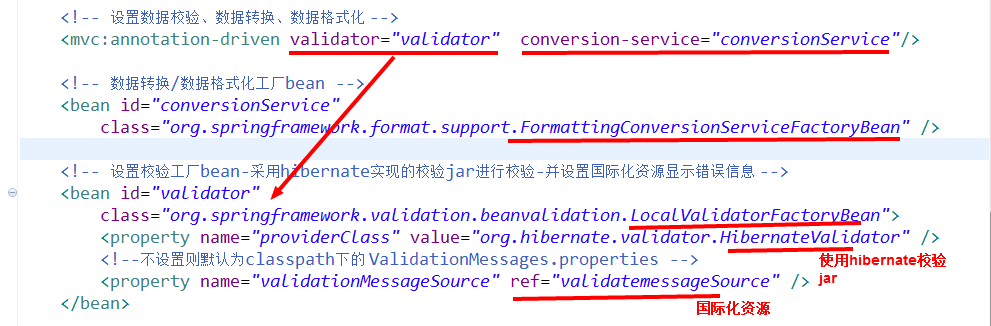

<?xml version="1.0" encoding="UTF-8" ?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:p="http://www.springframework.org/schema/p"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:mvc="http://www.springframework.org/schema/mvc"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans-3.0.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context-3.0.xsd
http://www.springframework.org/schema/mvc
http://www.springframework.org/schema/mvc/spring-mvc-3.0.xsd">
<!-- 扫描web包,应用Spring的注解 -->
<context:component-scan base-package="com.ll.web"/>
<!-- 配置视图解析器,将ModelAndView及字符串解析为具体的页面,默认优先级最低 -->
<bean
class="org.springframework.web.servlet.view.InternalResourceViewResolver"
p:viewClass="org.springframework.web.servlet.view.JstlView"
p:prefix="/jsp/"
p:suffix=".jsp" />
<!-- 设置数据校验、数据转换、数据格式化 -->
<mvc:annotation-driven validator="validator" conversion-service="conversionService"/>
<!-- 数据转换/数据格式化工厂bean -->
<bean id="conversionService"
class="org.springframework.format.support.FormattingConversionServiceFactoryBean" />
<!-- 设置校验工厂bean-采用hibernate实现的校验jar进行校验-并设置国际化资源显示错误信息 -->
<bean id="validator"
class="org.springframework.validation.beanvalidation.LocalValidatorFactoryBean">
<property name="providerClass" value="org.hibernate.validator.HibernateValidator" />
<!--不设置则默认为classpath下的 ValidationMessages.properties -->
<property name="validationMessageSource" ref="validatemessageSource" />
</bean>
<!-- 设置国际化资源显示错误信息 -->
<bean id="validatemessageSource"
class="org.springframework.context.support.ReloadableResourceBundleMessageSource">
<property name="basename">
<list>
<value>classpath:valideMessages</value>
</list>
</property>
<property name="fileEncodings" value="utf-8" />
<property name="cacheSeconds" value="120" />
</bean>
</beans>
3. 待校验的Java对象
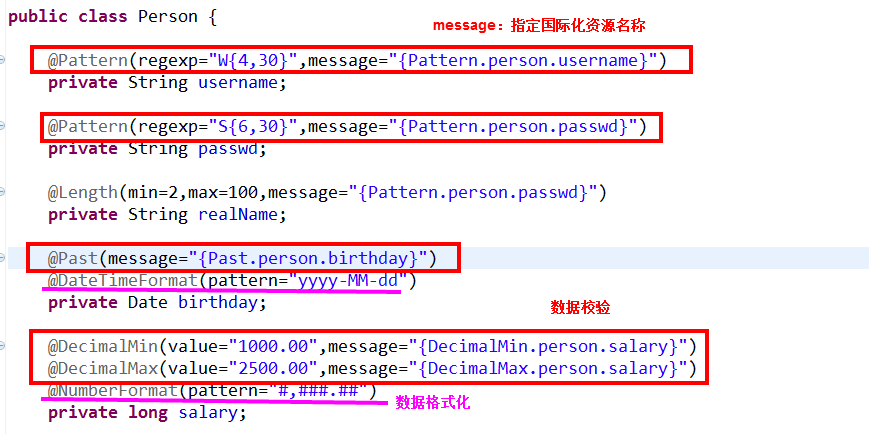
package com.ll.model;
import java.util.Date;
import javax.validation.constraints.DecimalMax;
import javax.validation.constraints.DecimalMin;
import javax.validation.constraints.Past;
import javax.validation.constraints.Pattern;
import org.hibernate.validator.constraints.Length;
import org.springframework.format.annotation.DateTimeFormat;
import org.springframework.format.annotation.NumberFormat;
public class Person {
@Pattern(regexp="W{4,30}",message="{Pattern.person.username}")
private String username;
@Pattern(regexp="S{6,30}",message="{Pattern.person.passwd}")
private String passwd;
@Length(min=2,max=100,message="{Pattern.person.passwd}")
private String realName;
@Past(message="{Past.person.birthday}")
@DateTimeFormat(pattern="yyyy-MM-dd")
private Date birthday;
@DecimalMin(value="1000.00",message="{DecimalMin.person.salary}")
@DecimalMax(value="2500.00",message="{DecimalMax.person.salary}")
@NumberFormat(pattern="#,###.##")
private long salary;
public Person() {
super();
}
public Person(String username, String passwd, String realName) {
super();
this.username = username;
this.passwd = passwd;
this.realName = realName;
}
public String getUsername() {
return username;
}
public void setUsername(String username) {
this.username = username;
}
public String getPasswd() {
return passwd;
}
public void setPasswd(String passwd) {
this.passwd = passwd;
}
public String getRealName() {
return realName;
}
public void setRealName(String realName) {
this.realName = realName;
}
public Date getBirthday() {
// DateFormat df = new SimpleDateFormat("yyyy-MM-dd");
// return df.format(birthday);
return birthday;
}
public void setBirthday(Date birthday) {
this.birthday = birthday;
}
public long getSalary() {
return salary;
}
public void setSalary(long salary) {
this.salary = salary;
}
@Override
public String toString() {
return "Person [username=" + username + ", passwd=" + passwd + "]";
}
}
4. 国际化资源文件



5. 控制层
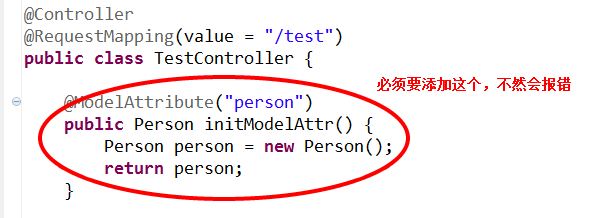
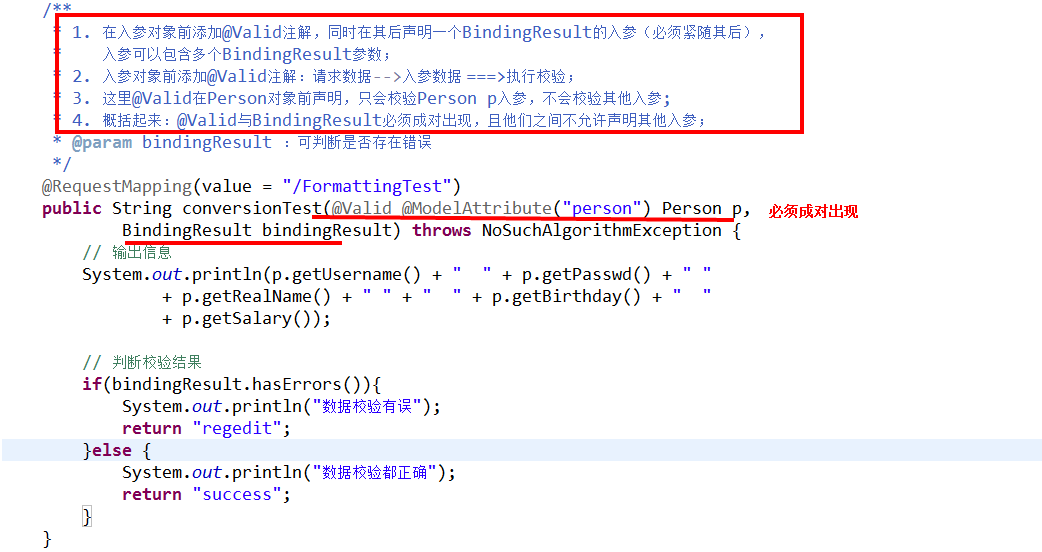
下面这张图是从其他文章中截取的,主要用于说明:@Valid与BindingResult之间的关系;
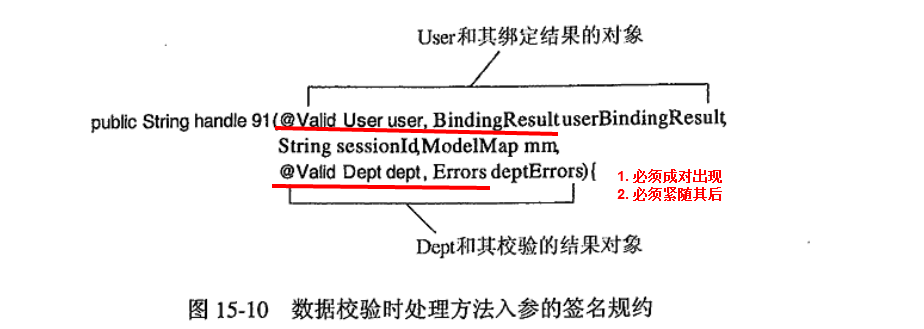
package com.ll.web;
import java.security.NoSuchAlgorithmException;
import javax.validation.Valid;
import org.springframework.stereotype.Controller;
import org.springframework.validation.BindingResult;
import org.springframework.web.bind.annotation.ModelAttribute;
import org.springframework.web.bind.annotation.RequestMapping;
import com.ll.model.Person;
/**
* @author Administrator
*
*/
@Controller
@RequestMapping(value = "/test")
public class TestController {
@ModelAttribute("person")
public Person initModelAttr() {
Person person = new Person();
return person;
}
/**
* 返回主页
* @return
*/
@RequestMapping(value = "/index.action")
public String index() {
return "regedit";
}
/**
* 1. 在入参对象前添加@Valid注解,同时在其后声明一个BindingResult的入参(必须紧随其后),
* 入参可以包含多个BindingResult参数;
* 2. 入参对象前添加@Valid注解:请求数据-->入参数据 ===>执行校验;
* 3. 这里@Valid在Person对象前声明,只会校验Person p入参,不会校验其他入参;
* 4. 概括起来:@Valid与BindingResult必须成对出现,且他们之间不允许声明其他入参;
* @param bindingResult :可判断是否存在错误
*/
@RequestMapping(value = "/FormattingTest")
public String conversionTest(@Valid @ModelAttribute("person") Person p,
BindingResult bindingResult) throws NoSuchAlgorithmException {
// 输出信息
System.out.println(p.getUsername() + " " + p.getPasswd() + " "
+ p.getRealName() + " " + " " + p.getBirthday() + " "
+ p.getSalary());
// 判断校验结果
if(bindingResult.hasErrors()){
System.out.println("数据校验有误");
return "regedit";
}else {
System.out.println("数据校验都正确");
return "success";
}
}
}
6. 前台
引入Spring的form标签:

在前台显示错误:
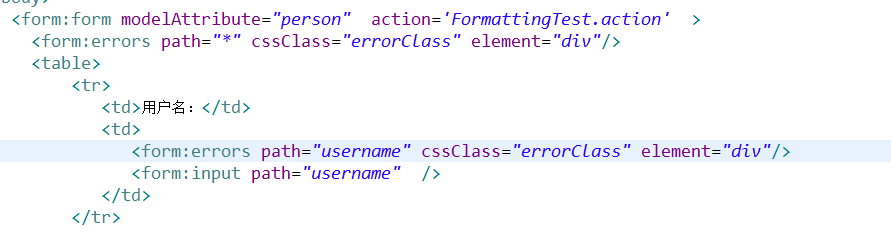
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%>
<%@ taglib prefix="form" uri="http://www.springframework.org/tags/form" %>
<html>
<head>
<title>数据校验</title>
<style>
.errorClass{color:red}
</style>
</head>
<body>
<form:form modelAttribute="person" action='FormattingTest.action' >
<form:errors path="*" cssClass="errorClass" element="div"/>
<table>
<tr>
<td>用户名:</td>
<td>
<form:errors path="username" cssClass="errorClass" element="div"/>
<form:input path="username" />
</td>
</tr>
<tr>
<td>密码:</td>
<td>
<form:errors path="passwd" cssClass="errorClass" element="div"/>
<form:password path="passwd" />
</td>
</tr>
<tr>
<td>真实名:</td>
<td>
<form:errors path="realName" cssClass="errorClass" element="div"/>
<form:input path="realName" />
</td>
</tr>
<tr>
<td>生日:</td>
<td>
<form:errors path="birthday" cssClass="errorClass" element="div"/>
<form:input path="birthday" />
</td>
</tr>
<tr>
<td>工资:</td>
<td>
<form:errors path="salary" cssClass="errorClass" element="div"/>
<form:input path="salary" />
</td>
</tr>
<tr>
<td colspan="2"><input type="submit" name="提交"/></td>
</tr>
</table>
</form:form>
</body>
</html>
7. 测试
输入:http://localhost:8080/SpringMVCTest/test/index.action
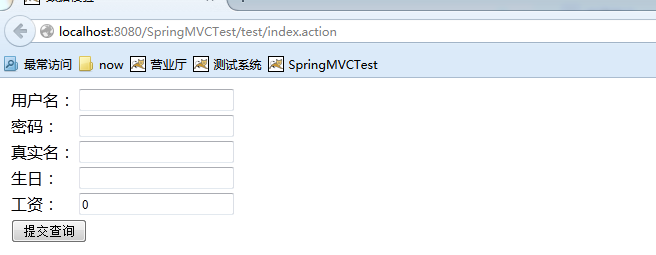
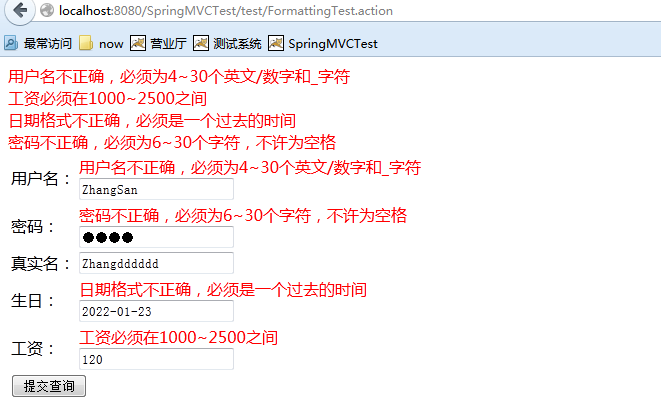

8. 其他
参考链接:
http://www.cnblogs.com/ssslinppp
http://blog.sina.com.cn/spstudy
http://www.cnblogs.com/liukemng/p/3738055.html
http://www.cnblogs.com/liukemng/p/3754211.html
淘宝源程序下载(可直接运行):
http://shop110473970.taobao.com/?spm=a230r.7195193.1997079397.42.AvYpGW
http://shop125186102.taobao.com/?spm=a1z10.1-c.0.0.SsuajD
来自为知笔记(Wiz)
附件列表